In this tutorial, you will learn to use symmetric cryptography in Java, you will learn the essential concepts of single key aka symmetric key cryptography for encryption and decryption using different symmetric algorithm like AES, DES, 3DES, Blowfish, and Twofish.
Symmetric Cryptography
- Symmetric cryptography use a single key for both encryption and decryption processes. The popular example of symmetric key cryptographic algorithm are AES, DES, 3DES, Blowfish, and Twofish.
- Symmetric cryptography, also known as secret-key cryptography, involves using a single key for both encryption and decryption processes.
- The key must be kept secret between the communicating parties to ensure the security of the data. Symmetric encryption is typically faster than asymmetric encryption, making it suitable for securing large volumes of data.
The primary components of symmetric key cryptography are:
- Plaintext: The original data that needs to be encrypted.
- Ciphertext: The encrypted data produced after applying the encryption algorithm with the secret key.
- Secret Key: The key used for both encryption and decryption processes.
- Encryption Algorithm: The mathematical algorithm used to convert plaintext into ciphertext.
- Decryption Algorithm: The mathematical algorithm used to convert ciphertext back into plaintext.
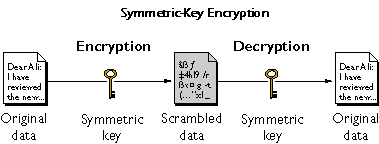
Symmetric key cryptography algorithms in java
AES (Advanced Encryption Standard)
- AES is a widely used symmetric encryption algorithm known for its security and efficiency.
- It supports key sizes of 128, 192, and 256 bits and operates on blocks of data.
- AES has replaced DES as the standard encryption algorithm due to its stronger security properties.
DES (Data Encryption Standard)
- DES is one of the earliest symmetric encryption algorithms developed by IBM in the 1970s.
- It operates on 64-bit blocks of data and uses a 56-bit key.
- However, DES is considered insecure for modern applications due to its small key size and vulnerability to brute-force attacks.
Triple DES (3DES)
- Triple DES, also known as 3DES or DESede, is an enhancement of the DES algorithm that applies DES encryption three times using different keys.
- It offers increased security compared to DES but is slower and less efficient due to multiple encryption rounds.
Blowfish
- Blowfish is a symmetric block cipher designed by Bruce Schneier in 1993.
- It supports variable key lengths from 32 bits to 448 bits and operates on 64-bit blocks of data.
- Blowfish is known for its simplicity and speed, making it suitable for various applications.
Twofish
- Twofish is a symmetric encryption algorithm developed as a candidate for the AES standard.
- It supports key sizes of 128, 192, and 256 bits and operates on 128-bit blocks of data.
- Twofish offers strong security and performance, making it a popular choice for encryption.
Mode of Operation in Symmetric key cryptography
Symmetric encryption algorithms operate in different modes to handle various data encryption scenarios. Common modes of operation include:
- ECB (Electronic Codebook):
- Encrypts each block of data independently, which can lead to security vulnerabilities due to patterns in the plaintext.
- CBC (Cipher Block Chaining):
- XORs each plaintext block with the previous ciphertext block before encryption, providing better security than ECB.
- CTR (Counter):
- Converts a block cipher into a stream cipher by encrypting a counter value, allowing parallel encryption and decryption.
Padding Schemes in Symmetric key cryptography
Padding schemes are used to ensure that the plaintext data fits into fixed-size blocks required by block ciphers. Common padding schemes include:
- PKCS#5/PKCS#7 Padding: Appends bytes to the plaintext to fill the last block, where each byte represents the number of padding bytes added.
- Zero Padding: Appends zero bytes to the plaintext to fill the last block.
- ISO/IEC 7816-4 Padding: Appends a single ’80’ byte followed by zero bytes to indicate the end of the plaintext.
Initialization vectors (IVs)
Initialization vectors (IVs) are used in some encryption modes, such as CBC, to ensure that each encrypted message produces a unique ciphertext even if the plaintext is the same. IVs should be random and unpredictable to enhance security.