In this tutorial you will learn to create a URL Shortener python project using sqlite3 database pyshorteners library.
1. Introduction
A URL shortener is a useful application that takes a long URL and converts it into a short URL. This tutorial involves building a URL shortener using Python, the pyshorteners
package, and an SQLite database.
2. Setting Up the Environment
2.1 Installing Pyshorteners
To get started, you need to install the pyshorteners
package. You can install it using pip:
pip install pyshorteners
2.2 Setting Up SQLite Database
We will use SQLite to store the original and shortened URLs. SQLite is a lightweight, disk-based database that doesn’t require a separate server process.
First, import the necessary libraries:
import pyshorteners
import sqlite3
3. Building the URL Shortener
3.1 Creating the Database Table
Next, we need to create a database and a table to store our URLs. The following code creates a database named urls.db
and a table named urls
if they don’t already exist:
# create database and table if they don't exist
conn = sqlite3.connect('urls.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS urls
(id INTEGER PRIMARY KEY AUTOINCREMENT, long_url TEXT, short_url TEXT)''')
conn.commit()
3.2 Shortening URLs
We can now create a function to shorten URLs using Pyshorteners and store them in the database:
def shorten_url(long_url):
s = pyshorteners.Shortener()
short_url = s.tinyurl.short(long_url)
# insert long and short URLs into database
c.execute("INSERT INTO urls (long_url, short_url) VALUES (?, ?)", (long_url, short_url))
conn.commit()
print(f'Short URL: {short_url}')
3.3 Listing All URLs
To view all the URLs stored in the database, we can create a function to list them:
def list_urls():
c.execute("SELECT long_url, short_url FROM urls")
rows = c.fetchall()
for row in rows:
print(f'{row[0]}: {row[1]}')
4. Running the Application
Finally, we can create a simple command-line interface to interact with our URL shortener:
while True:
print("Select an option:")
print("1. Shorten a URL")
print("2. List all URLs")
print("3. Quit")
choice = input()
if choice == "1":
long_url = input("Enter long URL: ")
shorten_url(long_url)
elif choice == "2":
list_urls()
elif choice == "3":
break
else:
print("Invalid choice")
With this code, you can run the application, shorten URLs, and list all the shortened URLs stored in your SQLite database.
Full Code Example for URL Shortener python project
Here is the complete code for your URL shortener application:
import pyshorteners
import sqlite3
# create database and table if they don't exist
conn = sqlite3.connect('urls.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS urls
(id INTEGER PRIMARY KEY AUTOINCREMENT, long_url TEXT, short_url TEXT)''')
conn.commit()
def shorten_url(long_url):
s = pyshorteners.Shortener()
short_url = s.tinyurl.short(long_url)
# insert long and short URLs into database
c.execute("INSERT INTO urls (long_url, short_url) VALUES (?, ?)", (long_url, short_url))
conn.commit()
print(f'Short URL: {short_url}')
def list_urls():
c.execute("SELECT long_url, short_url FROM urls")
rows = c.fetchall()
for row in rows:
print(f'{row[0]}: {row[1]}')
while True:
print("Select an option:")
print("1. Shorten a URL")
print("2. List all URLs")
print("3. Quit")
choice = input()
if choice == "1":
long_url = input("Enter long URL: ")
shorten_url(long_url)
elif choice == "2":
list_urls()
elif choice == "3":
break
else:
print("Invalid choice")
In the above Python project, we created a URL shortener command line project
using Python. We have used a Pyshorteners library
, and SQLite database
.
We have also added the option to list all the short URLs and their mappings. When you run the program, it will display a menu with three options:
- shorten a URL,
- list all URLs, and
- quit.
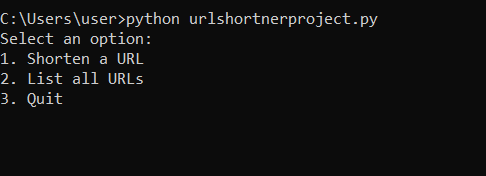
If you choose to shorten a URL, you will be prompted to enter the long URL. The program will then generate a short URL using the Pyshorteners library and insert both the long and short URLs into the SQLite database.
If you choose to list all URLs, the program will retrieve all the URLs and their mappings from the database and display them on the screen. If you choose to quit, the program will exit.