Python is a widely used, interpreted high-level programming language that is used for a wide range of tasks from machine learning to web development. Because of its ease of use and readability, it will be an excellent choice of language for beginner programmers. Real-world projects are one of the finest methods to learn the Python programming language. In this article, you will learn Python language by programming ten Python projects that will help you hone your skills and obtain real-world experience.
Top 10 python projects for Beginners to Start in 2024
Project 1: To-Do List App:
A to-do
list project is a simple Python project, that can help users to keep track of their tasks.
While programming this project you will learn to create Python functions to add
and remove
a task from a sqlite
database. You will also learn on using a while loop to continuously interact with the user and break a while loop when the user selects the exit menu.
The code for our Python todo-list
project is as follows.
import sqlite3
from prettytable import PrettyTable
# connect to the database
conn = sqlite3.connect('tasks.db')
# create a table for tasks
conn.execute('''
CREATE TABLE IF NOT EXISTS tasks
(id INTEGER PRIMARY KEY AUTOINCREMENT,
task TEXT NOT NULL,
status TEXT NOT NULL DEFAULT 'Incomplete')
''')
# define functions for database operations
def add_task(task):
conn.execute("INSERT INTO tasks (task) VALUES (?)", (task,))
conn.commit()
def delete_task(task_id):
conn.execute("DELETE FROM tasks WHERE id=?", (task_id,))
conn.commit()
def mark_task_complete(task_id):
conn.execute("UPDATE tasks SET status='Completed' WHERE id=?", (task_id,))
conn.commit()
def print_tasks():
tasks = conn.execute("SELECT * FROM tasks").fetchall()
table = PrettyTable()
table.field_names = ["ID", "Task", "Status"]
for task in tasks:
table.add_row([task[0], task[1], "(Completed)" if task[2]=="Completed" else ""])
print(table)
# main loop
while True:
print('1. Add Task')
print('2. Delete Task')
print('3. Mark Task as Completed')
print('4. Print Tasks')
print('5. Exit')
choice = input('Enter your choice: ')
if choice == '1':
task = input('Enter the task: ')
add_task(task)
elif choice == '2':
task_id = input('Enter the task ID to delete: ')
delete_task(task_id)
elif choice == '3':
task_id = input('Enter the task ID to mark as completed: ')
mark_task_complete(task_id)
elif choice == '4':
print_tasks()
elif choice == '5':
conn.close()
break
In the above project we begin by importing the required modules, sqlite3
for database operations and prettytable
for displaying tabular data in a user-friendly way.
After importing sqlite
we establish a connection to the SQLite database tasks.db
and create a table named tasks
with three columns: id, task, and status
. The id column is set to be the primary key and auto-incremented, so each task added to the table will be assigned a unique ID
.
After that, we define four functions for database operations. The add_task()
function takes a task as input and inserts it into the tasks table with a default status of “Incomplete“. The delete_task() function takes a task ID as input and deletes the corresponding task from the tasks table. The mark_task_complete() function takes a task ID as input and updates the status column of the corresponding task to “Completed“. Finally, the print_tasks() function retrieves all tasks from the tasks table, formats them into a pretty table using PrettyTable, and displays the table to the user.
In the while loop, we present the user with five options: add a task, delete a task, mark a task as completed, print the tasks, or exit the program. The user selects an option by entering a number, and the corresponding function is called.
- If the user selects option 1 to add a task, they are prompted to enter the task text.
- If the user selects option 2 or 3 to delete or mark a task, respectively, they are prompted to enter the ID of the task they want to modify.
- If the user selects option 4 to print the tasks, the print_tasks() function is called and a pretty table of all tasks with their IDs and statuses is displayed.
- If the user selects option 5 to exit the program, the database connection is closed and the loop is terminated.
Overall, this project demonstrates how to use SQLite databases and the prettytable module to create a simple command-line task manager.
The Output of todo app while running a project
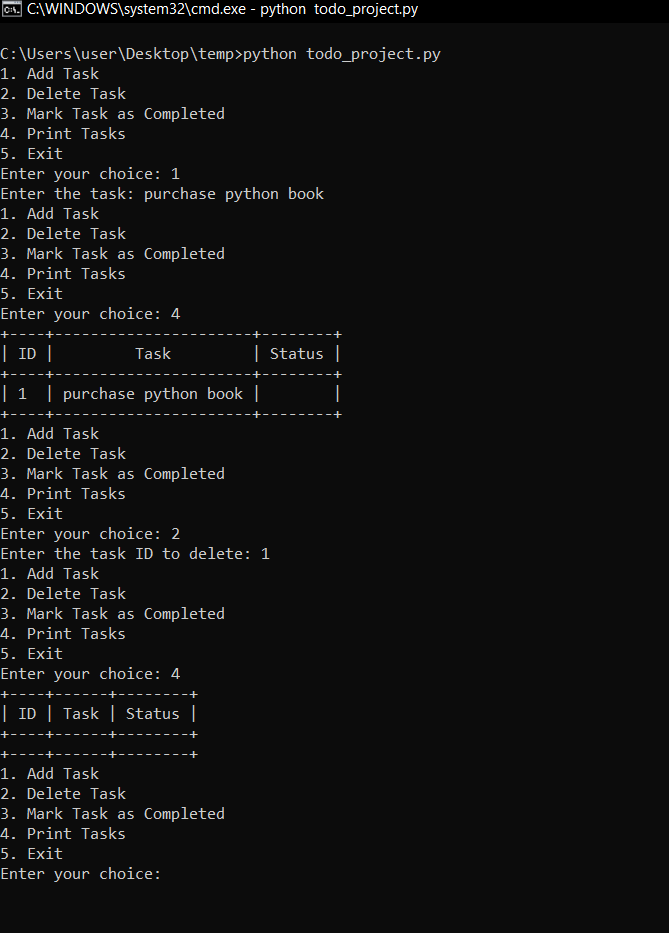
1. Add Task
2. Delete Task
3. Mark Task as Completed
4. Print Tasks
5. Exit
Enter your choice: 4
+----+-------------------+------------+
| ID | Task | Status |
+----+-------------------+------------+
| 1 | Do laundry | Completed |
| 2 | Buy groceries | Incomplete |
| 3 | Clean the house | Incomplete |
+----+-------------------+------------+
Enter your choice: 3
Enter the task ID to mark as completed: 2
Enter your choice: 4
+----+-------------------+------------+
| ID | Task | Status |
+----+-------------------+------------+
| 1 | Do laundry | Completed |
| 2 | Buy groceries | Completed |
| 3 | Clean the house | Incomplete |
+----+-------------------+------------+
Enter your choice: 2
Enter the task ID to delete: 3
Enter your choice: 5
What you have learned by building a Python todo project
Database operations: You will learn how to establish a connection, create a table, insert data, retrieve data, and update data in a database using Python. The project uses SQLite to create and interact with a database.
Using external libraries: You will learn how to install and import external libraries in your Python programs, as well as how to use their functions and methods. The project uses the sqlite3 and prettytable libraries to perform database operations and display data in a tabular format.
Command-line interface (CLI) programming: You will learn how to read user input, validate input, and display output in a CLI program. The project presents a simple CLI interface to the user, allowing them to add, delete, and mark tasks as complete, as well as view all tasks in a formatted table.
Debugging and error handling: You will learn how to debug your code and handle errors that may arise during execution, such as invalid input, missing data, or database errors. The project involves several functions that perform complex operations.
Project 2: A Calculator
In this project, you will learn to build a simple calculator in Python. You will use python functions
, while
loop and if-elif
python statement to build a calculator project.
This calculator that you will build is capable of performing basic arithmetic operations such as addition, subtraction, multiplication, and division as shown below.
from prettytable import PrettyTable
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
return x / y
while True:
print('Select operation.')
print('1. Add')
print('2. Subtract')
print('3. Multiply')
print('4. Divide')
print('5. Exit')
choice = input('Enter choice(1/2/3/4/5): ')
if choice == '5':
print('Exiting calculator')
break
num1 = float(input('Enter first number: '))
num2 = float(input('Enter second number: '))
table = PrettyTable(['Operation', 'Result'])
if choice == '1':
result = add(num1, num2)
table.add_row(['Add', result])
print(table)
elif choice == '2':
result = subtract(num1, num2)
table.add_row(['Subtract', result])
print(table)
elif choice == '3':
result = multiply(num1, num2)
table.add_row(['Multiply', result])
print(table)
elif choice == '4':
if num2 == 0:
print('Error: Cannot divide by zero')
else:
result = divide(num1, num2)
table.add_row(['Divide', result])
print(table)
else:
print('Invalid input')
The output of a calculator project
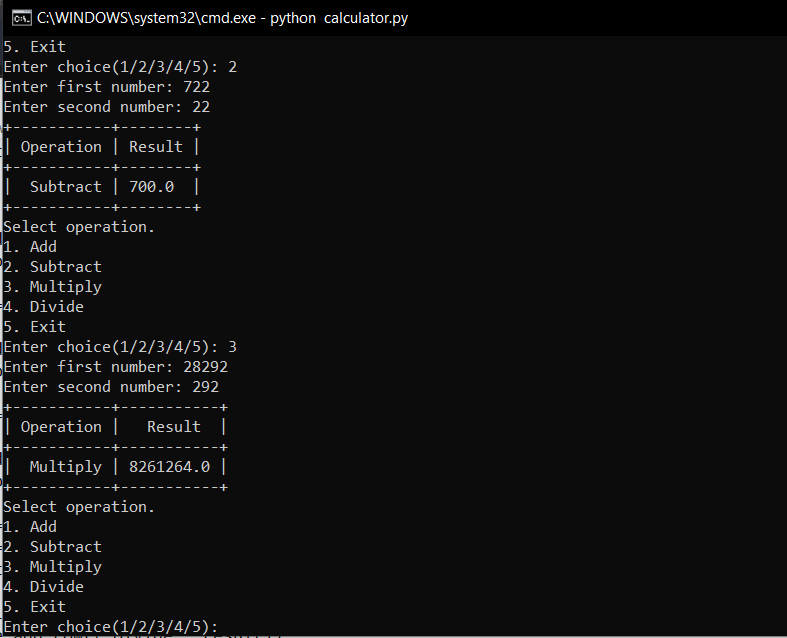
Project 3: A weather app
Another project that will be suited for a beginner is a weather app, it is a great project for beginners as it uses APIs to retrieve data from a remote server which makes the user familiar with using an API to fetch data from a remote server.
This weather app project that we are building displays the current weather conditions for a given city entered by a user.
In this project we are using the OpenWeatherMap API which provides weather data for different cities around the world. To use the API, we need to provide an API key that is unique to our account. We assign our API key to the api_key variable in the program below.
Here the user is asked to enter the name of the city for which they want to query the weather information once the user inputs the city name it is assigned to a city variable.
Next, A URL for the API endpoint is constructed by formatting the city and api_key variables into the URL string.
python requests
library is used to send the GET
request to the API endpoint using the requests.get()
function by passing in the URL as an argument.
The response
variable contains the server`s response to our request.
If the server response status code is 200
from the server it indicates a successful response, Once we get the successful response, we convert the response data to JSON format using the response.json()
function and assign it to the data variable. We then use the print function to print out the relevant weather information for the city, which includes the temperature, feels like the temperature, and weather description.
If the response status code is not 200, we print an error message indicating the status code and reason for the failure.
To configure an API key visit https://openweathermap.org/appid OpenWeatherMap link to grab your developer key which is so good and yet free.
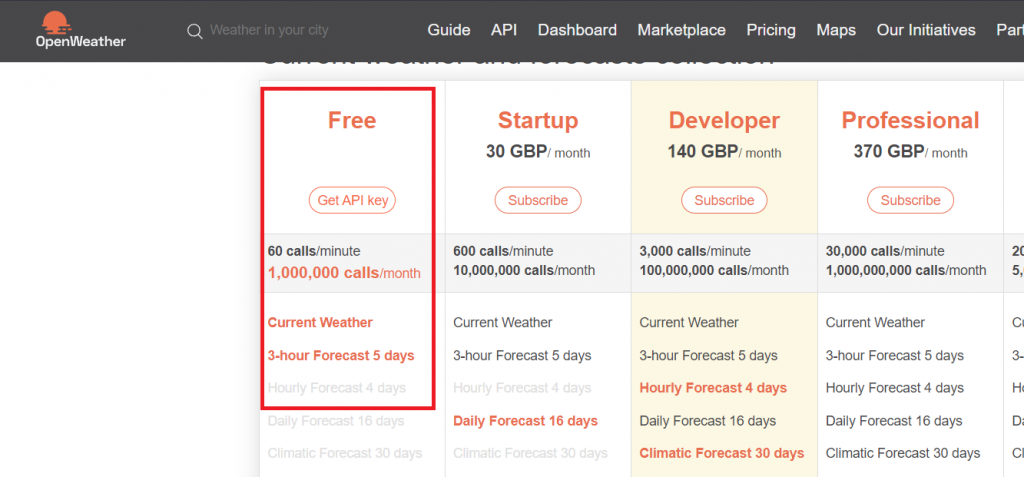
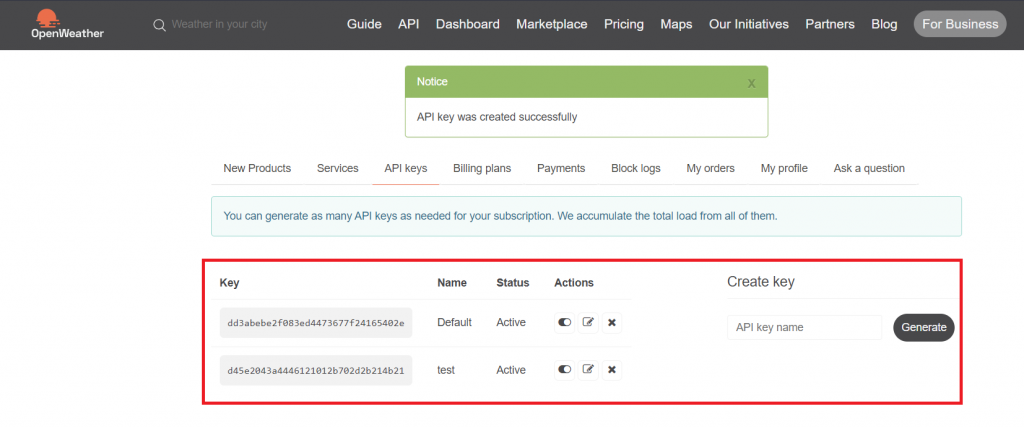
import requests
api_key = 'd45e2043a4446121012b702d2b214b21'
while True:
city = input('Enter city name (or type "exit" to quit): ')
if city.lower() == 'exit':
print('Exiting weather app')
break
url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}&units=metric'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(f"Weather information for {city}:")
print(f"Temperature: {data['main']['temp']}°C")
print(f"Feels like: {data['main']['feels_like']}°C")
print(f"Weather description: {data['weather'][0]['description']}")
else:
print(f"Error: {response.status_code} - {response.reason}")
The output of the Weather Project
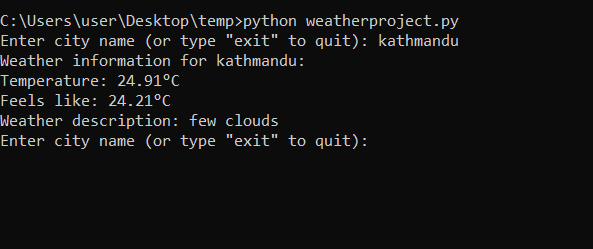
Project 4: Build a Snake Game:
If you want to create a classic game? With Python, you can build the beloved snake game! This beginner Python-based game project will teach you to move the snake on a screen, eat the food, and get bigger and bigger. To bring this game to life, you’ll need to employ PyGame, a Python game framework.
Below is the source code for the game.
import pygame, random
pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 600, 400
BLOCK_SIZE = 10
SNAKE_SPEED = 15
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Snake Game')
clock = pygame.time.Clock()
font = pygame.font.SysFont("bahnschrift", 25)
def draw_snake(snake_list):
for block in snake_list:
pygame.draw.rect(screen, (0, 0, 0), [block[0], block[1], BLOCK_SIZE, BLOCK_SIZE])
def message(text, color):
message = font.render(text, True, color)
screen.blit(message, [SCREEN_WIDTH / 6, SCREEN_HEIGHT / 3])
def game_loop():
game_over = False
x, y = SCREEN_WIDTH / 2, SCREEN_HEIGHT / 2
x_change, y_change = 0, 0
snake_list = []
snake_length = 1
foodx, foody = round(random.randrange(0, SCREEN_WIDTH - BLOCK_SIZE) / 10.0) * 10.0, round(random.randrange(0, SCREEN_HEIGHT - BLOCK_SIZE) / 10.0) * 10.0
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
x_change, y_change = -BLOCK_SIZE, 0
elif event.key == pygame.K_RIGHT:
x_change, y_change = BLOCK_SIZE, 0
elif event.key == pygame.K_UP:
x_change, y_change = 0, -BLOCK_SIZE
elif event.key == pygame.K_DOWN:
x_change, y_change = 0, BLOCK_SIZE
if x >= SCREEN_WIDTH or x < 0 or y >= SCREEN_HEIGHT or y < 0:
game_over = True
x += x_change
y += y_change
screen.fill((50, 153, 213))
pygame.draw.rect(screen, (0, 255, 0), [foodx, foody, BLOCK_SIZE, BLOCK_SIZE])
snake_head = []
snake_head.append(x)
snake_head.append(y)
snake_list.append(snake_head)
if len(snake_list) > snake_length:
del snake_list[0]
for block in snake_list[:-1]:
if block == snake_head:
game_over = True
draw_snake(snake_list)
pygame.display.update()
if x == foodx and y == foody:
foodx, foody = round(random.randrange(0, SCREEN_WIDTH - BLOCK_SIZE) / 10.0) * 10.0, round(random.randrange(0, SCREEN_HEIGHT - BLOCK_SIZE) / 10.0) * 10.0
snake_length += 1
clock.tick(SNAKE_SPEED)
pygame.quit()
quit()
game_loop()
The output of our snake game is below
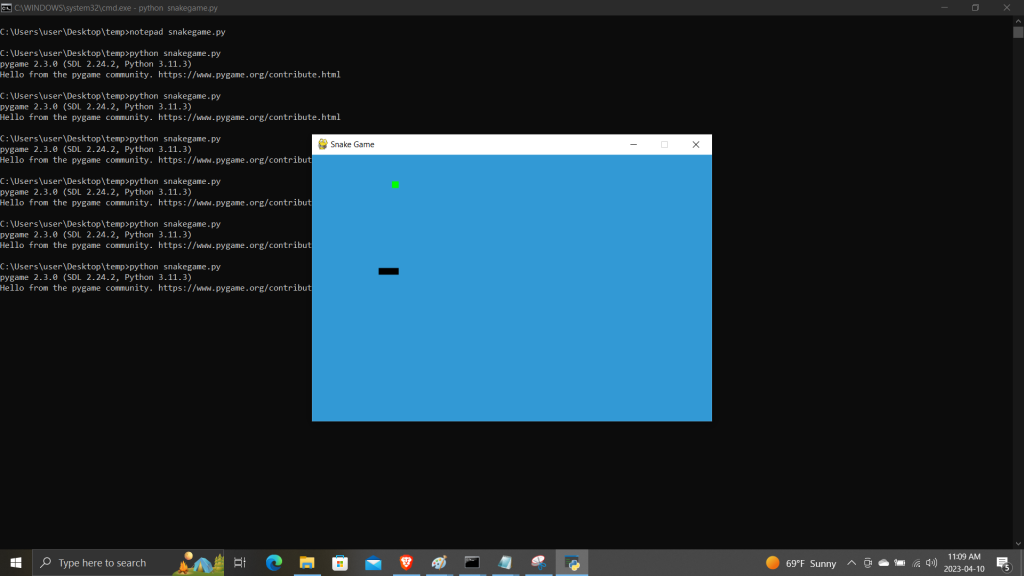
While building the snake in Python using, first we import the necessary libraries and initialize Pygame
. We set the screen size and block size of the Snake game. We also set the speed of the Snake, initialize the screen, and set the caption.
Next, we define a function to draw the Snake and another function to display messages on the screen. Then, we define the game loop, which starts by setting the game_over flag to False, initializing the position of the Snake, creating an empty list for the Snake, and randomly generating the position of the food.
Inside the game loop, we check for Pygame events such as the user closing the window or pressing a key. If the user presses an arrow key, we update the position of the Snake accordingly. If the Snake hits the boundaries of the screen or collides with itself, the game is over.
We update the position of the Snake, draw the Snake and the food on the screen, and check for collision with the food. If the Snake collides with the food, we generate a new position for the food and increase the length of the Snake.
Finally, we quit Pygame and end the program when the game is over.
Project 5: Create a Web Scraper:
Web scraping is a process of extracting content and data from a website using a bot or a program. Constructing a web scraper can be super helpful to automate tasks. By utilizing this skill, you can snatch data from websites and store it in a file to utilize at a later time.
In this web scraping project, you use a Python requests library and BeautifulSoup library to scrape country data from a Wikipedia page and store and print the result in a JSON format.
Before jumping into code make sure you have installed requests
and beauftifulsoup
library by running the command below.
pip install requests beautifulsoup4
import requests
from bs4 import BeautifulSoup
url = "https://en.wikipedia.org/wiki/List_of_countries_and_dependencies_by_population"
r = requests.get(url)
soup = BeautifulSoup(r.content)
table = soup.find("table", {"class": "wikitable sortable"})
data = []
rows = table.find_all("tr")
for row in rows[1:]:
cols = row.find_all("td")
if len(cols) > 1:
country = cols[1].text.strip()
population = cols[2].text.strip()
year = cols[3].text.strip()
data.append({"Country": country, "Population": population, "Year": year})
print(data)
The output of Python Web Scraper
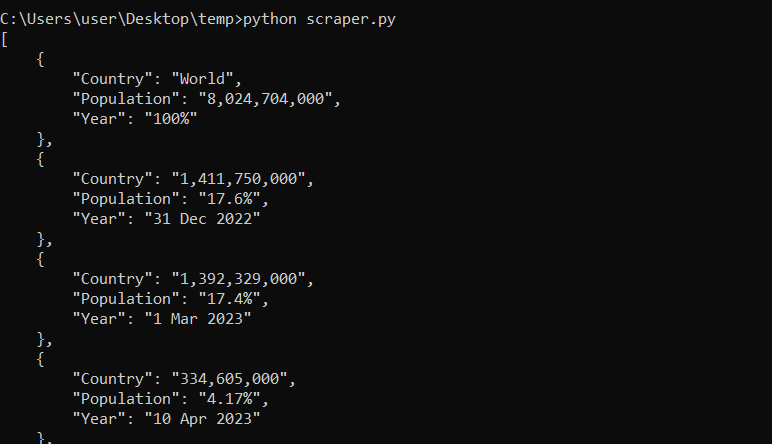
Project 6: Chatbot
As a beginner project, you can build a chatbot to learn a number of different Python concepts. A chatbot is a computer program that uses AI and NLP to understand user questions and respond to them by simulating human behavior in conversation. In this project, you build a chatbot using Python nltk a natural language processing library that can answer users’ questions on specific topics.
To run this project you need to install python nltk
library as shown below.
pip install nltk
from nltk.chat.util import Chat, reflections
QA = [
[
r"hi|hello",
["Hello there! How can I assist you today?", "Hi! How can I help you today?"]
],
[
r"what (is|are) your name ?",
["My name is Ada and I'm a chatbot. How about you?", ]
],
[
r"how (are you|do you do) ?",
["I'm doing well, thank you for asking. How about you?", "I'm a chatbot, so I don't have feelings like humans do. But I'm here to assist you!", ]
],
[
r"what (can|do) you (do|help with) ?",
["I can help you with a variety of things, such as answering your questions, providing information, or just having a chat. What can I help you with today?", ]
],
[
r"(.*) (created you|made you) ?",
["I was created by a team of developers who worked hard to bring me to life.", "My developers made me to help people like you. How can I assist you today?"]
],
[
r"what (is|are) your (specialty|expertise) ?",
["My expertise lies in providing assistance and answering your questions to the best of my abilities. What can I help you with today?"]
],
[
r"(.*) (location|city) ?",
["I don't have a physical location, as I'm a chatbot. But I'm here to help you with whatever you need!", ]
],
[
r"(.*) (weather|temperature) (in|at) (.*)?",
["You can check the weather at %4 by visiting a weather website or app. Is there anything else I can help you with?", "I'm not sure about the weather at %4, but you can check online to find out. What else can I assist you with today?"]
],
[
r"what (is|are) your (hobbies|interests) ?",
["As a chatbot, I don't have hobbies or interests like humans do. However, I'm always interested in helping you with whatever you need. What can I assist you with today?", ]
],
[
r"(.*) (thanks|thank you) (.*)",
["You're welcome! Is there anything else I can help you with?", "No problem, happy to help. What else can I assist you with today?"]
],
[
r"(.*) (bye|goodbye) (.*)",
["Goodbye! It was nice chatting with you. If you have any more questions or need assistance in the future, don't hesitate to come back and chat with me again.", "Take care, and have a great day!"]
],
[
r"(.*)",
["I'm sorry, I don't quite understand. Can you please rephrase your question or request?", "I'm not sure what you're asking. Can you please clarify or provide more information?"]
]
]
def ada():
print("Hi, I'm Ada. How can I assist you today? \nPlease type lowercase English language to start a conversation. Type 'bye' to end the conversation.")
chat = Chat(QA, reflections)
if __name__ == "__main__":
ada()
chat.converse()
Output
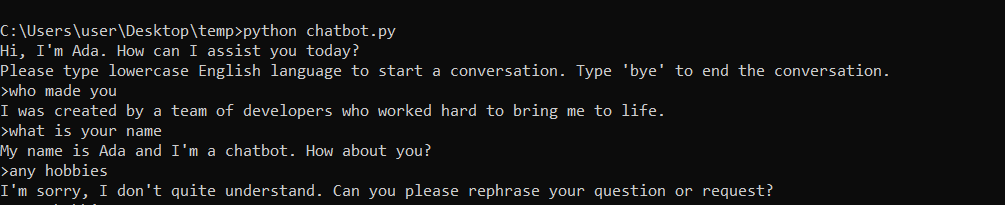
In the above chatbot program, we use the Python NLTK
library to engage in conversation with the user. When you run the program, the chatbot greets you and invites you to start chatting in lowercase English language.
Once you type in a message, the program searches for a matching question from a predefined list and returns an answer.
The chatbot is capable of handling different types of questions such as those related to weather or directions, thanks to the conditional statements in the code.
When you are ready to end the conversation, simply type “quit” and the chatbot will say goodbye.
To ensure that the program only runs when executed directly as a script, we have used the if name == “main“: block. Overall, for a beginner to learn pythons this project provides an opportunity of how NLTK can be used to create a simple chatbot that can respond to user input in a conversational manner.
Project 7: A URL Shortener
A URL shortener is a useful application that can be built using Python. This project involves building a URL shortener that takes a long URL and converts it to a short URL. To complete this project you need to install `pyshorteners` package and sqlite database
import pyshorteners
import sqlite3
# create database and table if they don't exist
conn = sqlite3.connect('urls.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS urls
(id INTEGER PRIMARY KEY AUTOINCREMENT, long_url TEXT, short_url TEXT)''')
conn.commit()
def shorten_url(long_url):
s = pyshorteners.Shortener()
short_url = s.tinyurl.short(long_url)
# insert long and short URLs into database
c.execute("INSERT INTO urls (long_url, short_url) VALUES (?, ?)", (long_url, short_url))
conn.commit()
print(f'Short URL: {short_url}')
def list_urls():
c.execute("SELECT long_url, short_url FROM urls")
rows = c.fetchall()
for row in rows:
print(f'{row[0]}: {row[1]}')
while True:
print("Select an option:")
print("1. Shorten a URL")
print("2. List all URLs")
print("3. Quit")
choice = input()
if choice == "1":
long_url = input("Enter long URL: ")
shorten_url(long_url)
elif choice == "2":
list_urls()
elif choice == "3":
break
else:
print("Invalid choice")
In the above Python project, we created a URL shortener command line project
using Python. We have used a Pyshorteners library
, and SQLite database
.
We have also added the option to list all the short URLs and their mappings. When you run the program, it will display a menu with three options:
- shorten a URL,
- list all URLs, and
- quit.
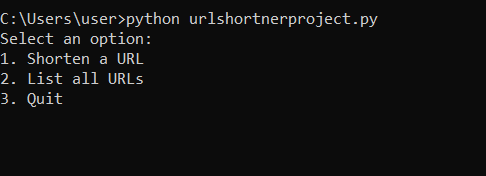
If you choose to shorten a URL, you will be prompted to enter the long URL. The program will then generate a short URL using the Pyshorteners library and insert both the long and short URLs into the SQLite database.
If you choose to list all URLs, the program will retrieve all the URLs and their mappings from the database and display them on the screen. If you choose to quit, the program will exit.
Project 8: Currency Converter
A currency converter is a useful project for beginners to learn Python.
In this currency converter project, we use the exchange rate API to convert currency from one currency to another. The project uses the requests module to make a GET request to the API URL and obtain the JSON response.
The program then extracts the rates dictionary from the JSON response, prompting the user to enter the currency codes for the currencies they want to convert and the amount to convert.
The program uses these values and the rates dictionary to compute the converted amount and display the result to the user. The program has a menu option to list all the available currency rates for the USD base currency. This project involves building a currency converter that converts one currency to another based on the current exchange rates.
import requests
url = 'https://api.exchangerate-api.com/v4/latest/USD'
response = requests.get(url)
data = response.json()
rates = data['rates']
def convert_currency():
currency1 = input('Enter currency to convert from: ')
currency2 = input('Enter currency to convert to: ')
amount = float(input('Enter amount to convert: '))
result = amount * (rates[currency2] / rates[currency1])
print(f'{amount} {currency1} is equal to {result} {currency2}')
def list_rates():
for currency, rate in rates.items():
print(f'{currency}: {rate}')
while True:
print("Select an option:")
print("1. Convert currency")
print("2. List all exchange rates")
print("3. Quit")
choice = input()
if choice == "1":
convert_currency()
elif choice == "2":
list_rates()
elif choice == "3":
break
else:
print("Invalid choice")
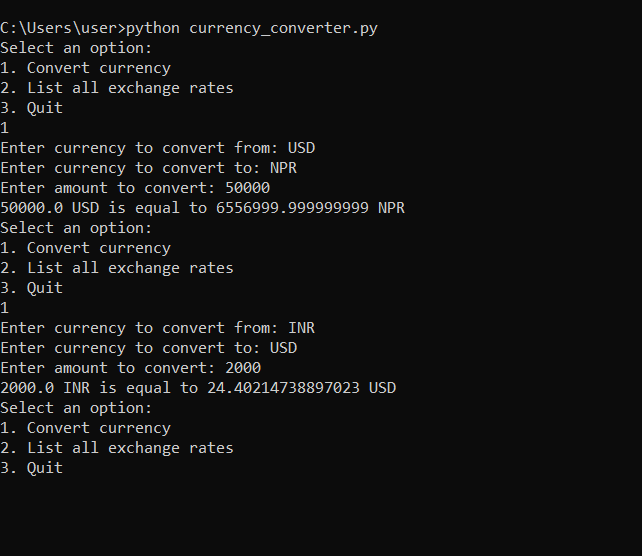
Project 9: Password Manager
A password manager is a useful application that can be built using Python. In this project, you will build a password manager that allows users to securely store and manage their passwords of different websites.
import secrets
import hashlib
import hmac
import sqlite3
from prettytable import PrettyTable
# Connect to the database
conn = sqlite3.connect('passwords.db')
# Create a table to store the passwords
conn.execute('''CREATE TABLE IF NOT EXISTS passwords
(website TEXT NOT NULL, username TEXT NOT NULL, password BLOB NOT NULL);''')
conn.commit()
# Function to encrypt a password
def encrypt_password(password):
salt = secrets.token_bytes(16)
key = hashlib.pbkdf2_hmac('sha256', password.encode('utf-8'), salt, 100000)
return salt + key
# Function to verify a password
def verify_password(password, hashed_password):
salt = hashed_password[:16]
key = hashed_password[16:]
new_key = hashlib.pbkdf2_hmac('sha256', password.encode('utf-8'), salt, 100000)
return hmac.compare_digest(key, new_key)
# Function to add a new password to the database
def add_password():
website = input('Enter website name: ')
username = input('Enter username: ')
password = input('Enter password: ')
hashed_password = encrypt_password(password)
conn.execute("INSERT INTO passwords (website, username, password) VALUES (?, ?, ?)", (website, username, hashed_password))
conn.commit()
print('Password added successfully.')
# Function to retrieve a password from the database
def get_password():
website = input('Enter website name: ')
password = input('Enter password: ')
cursor = conn.execute("SELECT username, password FROM passwords WHERE website=?", (website,))
row = cursor.fetchone()
if row:
username = row[0]
hashed_password = row[1]
if verify_password(password, hashed_password):
print(f'Website: {website}')
print(f'Username: {username}')
print(f'Password: {password}')
else:
print('Invalid password.')
else:
print('Website not found.')
# Function to list all passwords in the database
def list_passwords():
cursor = conn.execute("SELECT website, username, password FROM passwords")
rows = cursor.fetchall()
if len(rows) > 0:
table = PrettyTable(['Website', 'Username', 'Password'])
for row in rows:
website = row[0]
username = row[1]
password = row[2]
table.add_row([website, username, password])
print(table)
else:
print('No passwords found.')
# Main menu loop
while True:
print('1. Add Password')
print('2. Retrieve Password')
print('3. List Passwords')
print('4. Exit')
choice = input('Enter choice: ')
if choice == '1':
add_password()
elif choice == '2':
get_password()
elif choice == '3':
list_passwords()
elif choice == '4':
break
else:
print('Invalid choice. Try again.')
Output while running above program is shown below.
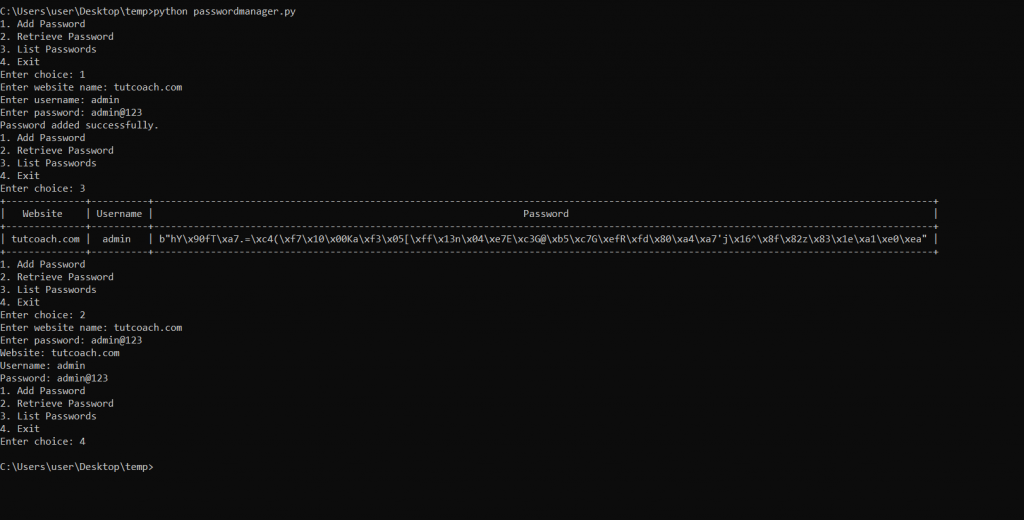
The above password manager project uses the following packages: secrets, hashlib, hmac, and sqlite3
.
The secrets package used here is used to generate a random salt that we use to hash the user’s password using the hashlib.pbkdf2_hmac
function. We also use the hmac
package to compare the hashed password with the user’s input.
We have also used the prettytable
information in tabular format for our users.
To store the passwords securely, we use an SQLite database to encrypt and decrypt the passwords. We create a table called passwords
that has three columns: website, username, and password.
We use the sqlite3 package to create and connect to the database and execute SQL queries.
The program allows the user to add a new password
, list all passwords, and retrieve a password
by website name. We use the input function to get the user’s input and the print function to display messages to the user. We also use the format function to format the output of the list_passwords function in a tabular format.
Overall, the program provides a secure and convenient way to manage passwords for various websites.
Project 10: Hangman Game
Hangman is a classic word-guessing game that can be built using Python. In this project, you will create a game that randomly selects a word from a list and asks the player to guess the letters in the word. The player has a limited number of attempts to guess the word before they lose the game.
To start, create a list of words that the game can select from. You can use a text file that contains a list of words or define the list of words in your code. Next, generate a random word from the list of words using the random
module.
Then, create a loop that runs until the player has used up all their attempts or has guessed the word correctly. Inside the loop, ask the player to guess a letter and check if the letter is in the word. If the letter is in the word, update a list that shows the letters that the player has correctly guessed. If the letter is not in the word, decrease the number of attempts remaining.
import random
words = ['apple', 'java']
def get_word():
random_word = random.choice(words)
random_word = list(random_word.upper())
return random_word
def draw_hangman(tries):
hangmanpics = ['''
+---+
| |
|
|
|
|
=========''', '''
+---+
| |
O |
|
|
|
=========''', '''
+---+
| |
O |
| |
|
|
=========''', '''
+---+
| |
O |
/| |
|
|
=========''', '''
+---+
| |
O |
/|\ |
|
|
=========''', '''
+---+
| |
O |
/|\ |
/ |
|
=========''', '''
+---+
| |
O |
/|\ |
/ \ |
|
=========''']
print(hangmanpics[tries])
game_word = get_word()
guessed_word = ['_'] * len(game_word)
tries = 0
# Loop for menu
while True:
# Print menu
print("Welcome to Python Hangman Game!")
print("1. Start a new game")
print("2. Exit game")
# Get user input for menu choice
choice = input("Enter your choice: ")
# Check if choice is valid
if choice not in ['1', '2']:
print("Invalid choice. Please try again.")
continue
# Exit game
if choice == '2':
print("Goodbye!")
break
# Start new game
game_word = get_word()
guessed_word = ['_'] * len(game_word)
tries = 0
# Print initial game state
print("=" * 100)
draw_hangman(tries)
print("Guess this word: ", " ".join(guessed_word))
# Play game
while True:
# Ask for user input
guess = input("Guess a letter: ").upper()
if len(guess) != 1 or not guess.isalpha():
print("Invalid input. Please enter a single letter.")
continue
if guess not in game_word:
print("Incorrect guess.")
tries += 1
draw_hangman(tries)
if tries > 6:
print("You lost the game. The word was:", "".join(game_word))
break
elif guess in game_word:
if guess in guessed_word:
print("You have already guessed this letter. Try another.")
continue
else:
print("Good job!")
for index, letter in enumerate(game_word):
if letter == guess:
guessed_word[index] = letter
print("Word: ", " ".join(guessed_word))
if guessed_word == game_word:
print("Congratulations, you won!")
break
The Demo of the running project is shown below.
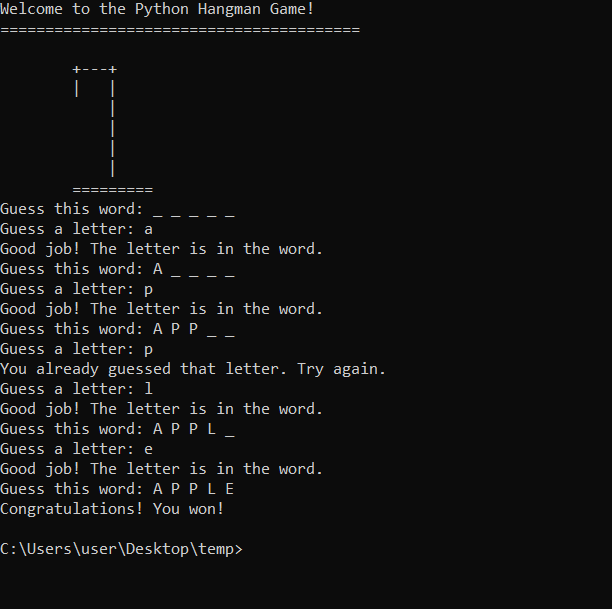
To complete this project First, we need to import a module called “random“. This module will help us choose a random word from a list of words that we will provide. We can add as many words as we want to this list, but for now, we only have two words: “apple” and “java”.
Next, we define two functions: “get_word()” and “draw_hangman(tries)”. The “get_word()” function will choose a random word from our list and convert it to uppercase letters. It will then return this word as a list of letters. The “draw_hangman(tries)” function will help us draw the hangman on the screen based on the number of tries we have.
After we define our functions, we create some variables to keep track of our game progress. We set the game_word
variable to the result of calling the get_word()
function. We set the guessed_word
variable to a list of underscores, one for each letter in the game_word
. We also set the tries
variable to 0 to keep track of how many tries we’ve had so far.
Next, we print some information about the game, including the hangman in its initial state. Then we print the current progress of the game using the guessed_word
variable.
Now, we start a “while” loop that will continue running until the game is won or lost. In each iteration of the loop, we ask the user to guess a letter. If the user’s input is not a single letter or is not a letter at all, we tell them it’s an invalid character and ask them to try again. If the user’s guess is not in the “game_word”, we tell them it’s an incorrect guess, and we increase the “tries” variable. Then we call the “draw_hangman(tries)” function to draw the next part of the hangman based on the number of tries.
If the user’s guess is in the “game_word”, we check if it’s already been guessed before. If it has, we tell the user to try another letter. If it hasn’t been guessed before, we congratulate the user and update the “guessed_word” variable to reflect the new guess. We also print the current progress of the game using the “guessed_word” variable.
Finally, if the “guessed_word” variable is the same as the “game_word” variable, we tell the user that they won the game and break out of the “while” loop. If the “tries” variable is greater than 6, we tell the user that they lost the game and break out of the “while” loop.
So that’s it! We can now play Hangman and have fun guessing words before our character, the Hangman, is completely drawn.
Conclusion
We hope that this article has been helpful in expanding your Python skills through the creation of ten different projects. By working on projects such as a Python todo list app, calculator, weather app, web scraper, snake game, chatbot, currency converter, URL shortener, hangman, and password manager, you have gained experience in a variety of Python concepts that can be applied to many other projects. We encourage you to continue practicing and learning through coding projects to further develop your skills.