In this tutorial you will learn to create a snake game in python.
If you want to create a classic game? With Python, you can build the beloved snake game! This beginner Python-based game project will teach you to move the snake on a screen, eat the food, and get bigger and bigger. To bring this game to life, you’ll need to employ PyGame, a Python game framework.
Below is the source code for the game.
import pygame, random
pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 600, 400
BLOCK_SIZE = 10
SNAKE_SPEED = 15
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Snake Game')
clock = pygame.time.Clock()
font = pygame.font.SysFont("bahnschrift", 25)
def draw_snake(snake_list):
for block in snake_list:
pygame.draw.rect(screen, (0, 0, 0), [block[0], block[1], BLOCK_SIZE, BLOCK_SIZE])
def message(text, color):
message = font.render(text, True, color)
screen.blit(message, [SCREEN_WIDTH / 6, SCREEN_HEIGHT / 3])
def game_loop():
game_over = False
x, y = SCREEN_WIDTH / 2, SCREEN_HEIGHT / 2
x_change, y_change = 0, 0
snake_list = []
snake_length = 1
foodx, foody = round(random.randrange(0, SCREEN_WIDTH - BLOCK_SIZE) / 10.0) * 10.0, round(random.randrange(0, SCREEN_HEIGHT - BLOCK_SIZE) / 10.0) * 10.0
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
x_change, y_change = -BLOCK_SIZE, 0
elif event.key == pygame.K_RIGHT:
x_change, y_change = BLOCK_SIZE, 0
elif event.key == pygame.K_UP:
x_change, y_change = 0, -BLOCK_SIZE
elif event.key == pygame.K_DOWN:
x_change, y_change = 0, BLOCK_SIZE
if x >= SCREEN_WIDTH or x < 0 or y >= SCREEN_HEIGHT or y < 0:
game_over = True
x += x_change
y += y_change
screen.fill((50, 153, 213))
pygame.draw.rect(screen, (0, 255, 0), [foodx, foody, BLOCK_SIZE, BLOCK_SIZE])
snake_head = []
snake_head.append(x)
snake_head.append(y)
snake_list.append(snake_head)
if len(snake_list) > snake_length:
del snake_list[0]
for block in snake_list[:-1]:
if block == snake_head:
game_over = True
draw_snake(snake_list)
pygame.display.update()
if x == foodx and y == foody:
foodx, foody = round(random.randrange(0, SCREEN_WIDTH - BLOCK_SIZE) / 10.0) * 10.0, round(random.randrange(0, SCREEN_HEIGHT - BLOCK_SIZE) / 10.0) * 10.0
snake_length += 1
clock.tick(SNAKE_SPEED)
pygame.quit()
quit()
game_loop()
The output of our snake game is below
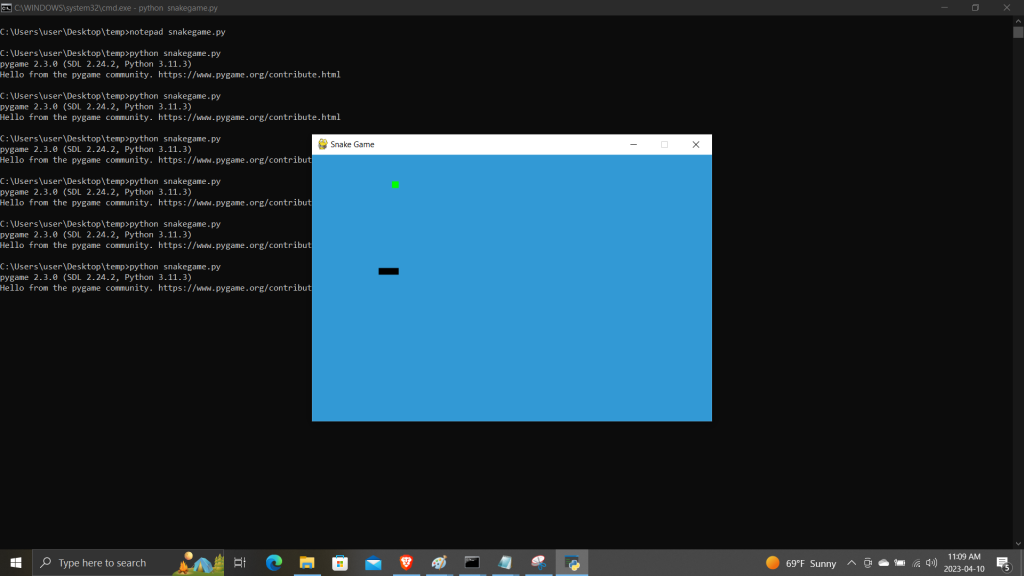
While building the snake in Python using, first we import the necessary libraries and initialize Pygame
. We set the screen size and block size of the Snake game. We also set the speed of the Snake, initialize the screen, and set the caption.
Next, we define a function to draw the Snake and another function to display messages on the screen. Then, we define the game loop, which starts by setting the game_over flag to False, initializing the position of the Snake, creating an empty list for the Snake, and randomly generating the position of the food.
Inside the game loop, we check for Pygame events such as the user closing the window or pressing a key. If the user presses an arrow key, we update the position of the Snake accordingly. If the Snake hits the boundaries of the screen or collides with itself, the game is over.
We update the position of the Snake, draw the Snake and the food on the screen, and check for collision with the food. If the Snake collides with the food, we generate a new position for the food and increase the length of the Snake.
Finally, we quit Pygame and end the program when the game is o