Creating a Python to do app project is a great way to dive into Python programming, as it combines fundamental concepts with practical application development. In this article, we’ll walk through the process of building a Python-based to-do app from scratch, covering key concepts such as data management, command line based user interface design, and task prioritization.
While programming this project, you’ll learn how to create Python functions that add and remove tasks from a SQLite database, as well as how to use a while loop to continuously interact with the user, breaking the loop only when the user selects the exit menu.
The code for our Python todo-list
project is as follows.
import sqlite3
from prettytable import PrettyTable
# connect to the database
conn = sqlite3.connect('tasks.db')
# create a table for tasks
conn.execute('''
CREATE TABLE IF NOT EXISTS tasks
(id INTEGER PRIMARY KEY AUTOINCREMENT,
task TEXT NOT NULL,
status TEXT NOT NULL DEFAULT 'Incomplete')
''')
# define functions for database operations
def add_task(task):
conn.execute("INSERT INTO tasks (task) VALUES (?)", (task,))
conn.commit()
def delete_task(task_id):
conn.execute("DELETE FROM tasks WHERE id=?", (task_id,))
conn.commit()
def mark_task_complete(task_id):
conn.execute("UPDATE tasks SET status='Completed' WHERE id=?", (task_id,))
conn.commit()
def print_tasks():
tasks = conn.execute("SELECT * FROM tasks").fetchall()
table = PrettyTable()
table.field_names = ["ID", "Task", "Status"]
for task in tasks:
table.add_row([task[0], task[1], "(Completed)" if task[2]=="Completed" else ""])
print(table)
# main loop
while True:
print('1. Add Task')
print('2. Delete Task')
print('3. Mark Task as Completed')
print('4. Print Tasks')
print('5. Exit')
choice = input('Enter your choice: ')
if choice == '1':
task = input('Enter the task: ')
add_task(task)
elif choice == '2':
task_id = input('Enter the task ID to delete: ')
delete_task(task_id)
elif choice == '3':
task_id = input('Enter the task ID to mark as completed: ')
mark_task_complete(task_id)
elif choice == '4':
print_tasks()
elif choice == '5':
conn.close()
break
In the project above, we start by importing necessary modules such as sqlite3
for database operations and prettytable
for presenting data in a user-friendly format.
Following the import of SQLite, we establish a connection to a database named tasks.db
and create a table named tasks
with columns for id
, task
, and status
. The id
column serves as the primary key and auto-increments to assign a unique ID to each task added.
We then define four functions for interacting with the database:
add_task()
inserts a new task with a default “Incomplete” status.delete_task()
removes a task based on its ID.mark_task_complete()
updates a task’s status to “Completed” based on its ID.print_tasks()
retrieves all tasks from the database, formats them into a readable table using PrettyTable, and displays them to the user.
Within the while loop, the user is presented with five options: add a task, delete a task, mark a task as completed, print tasks, or exit the program. The user selects an option by entering a corresponding number, triggering the respective function.
- Option 1 prompts the user to input a new task.
- Options 2 and 3 require the user to input the ID of the task they want to delete or mark as complete.
- Option 4 invokes the
print_tasks()
function to display all tasks in a formatted table. - Option 5 closes the database connection and ends the loop, exiting the program.
The Output of todo app while running a project
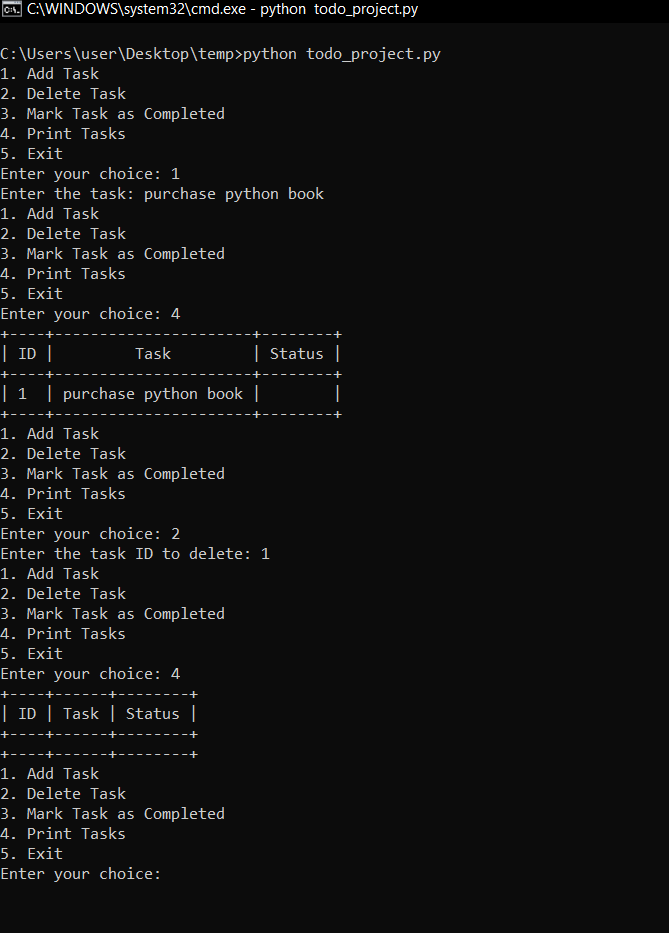
1. Add Task
2. Delete Task
3. Mark Task as Completed
4. Print Tasks
5. Exit
Enter your choice: 4
+----+-------------------+------------+
| ID | Task | Status |
+----+-------------------+------------+
| 1 | Do laundry | Completed |
| 2 | Buy groceries | Incomplete |
| 3 | Clean the house | Incomplete |
+----+-------------------+------------+
Enter your choice: 3
Enter the task ID to mark as completed: 2
Enter your choice: 4
+----+-------------------+------------+
| ID | Task | Status |
+----+-------------------+------------+
| 1 | Do laundry | Completed |
| 2 | Buy groceries | Completed |
| 3 | Clean the house | Incomplete |
+----+-------------------+------------+
Enter your choice: 2
Enter the task ID to delete: 3
Enter your choice: 5
What you have learned by building a Python todo project
Database operations: You will learn how to establish a connection, create a table, insert data, retrieve data, and update data in a database using Python. The project uses SQLite to create and interact with a database.
Using external libraries: You will learn how to install and import external libraries in your Python programs, as well as how to use their functions and methods. The project uses the sqlite3 and prettytable libraries to perform database operations and display data in a tabular format.
Command-line interface (CLI) programming: You will learn how to read user input, validate input, and display output in a CLI program. The project presents a simple CLI interface to the user, allowing them to add, delete, and mark tasks as complete, as well as view all tasks in a formatted table.
Debugging and error handling: You will learn how to debug your code and handle errors that may arise during execution, such as invalid input, missing data, or database errors. The project involves several functions that perform complex operations.