In this tutorial you will learn to create a python chatbot project using ntlk python library.
As a beginner project, you can build a chatbot to learn a number of different Python concepts. A chatbot is a computer program that uses AI and NLP to understand user questions and respond to them by simulating human behavior in conversation. In this project, you build a chatbot using Python nltk a natural language processing library that can answer users’ questions on specific topics.
To run this project you need to install python nltk
library as shown below.
pip install nltk
from nltk.chat.util import Chat, reflections
QA = [
[
r"hi|hello",
["Hello there! How can I assist you today?", "Hi! How can I help you today?"]
],
[
r"what (is|are) your name ?",
["My name is Ada and I'm a chatbot. How about you?", ]
],
[
r"how (are you|do you do) ?",
["I'm doing well, thank you for asking. How about you?", "I'm a chatbot, so I don't have feelings like humans do. But I'm here to assist you!", ]
],
[
r"what (can|do) you (do|help with) ?",
["I can help you with a variety of things, such as answering your questions, providing information, or just having a chat. What can I help you with today?", ]
],
[
r"(.*) (created you|made you) ?",
["I was created by a team of developers who worked hard to bring me to life.", "My developers made me to help people like you. How can I assist you today?"]
],
[
r"what (is|are) your (specialty|expertise) ?",
["My expertise lies in providing assistance and answering your questions to the best of my abilities. What can I help you with today?"]
],
[
r"(.*) (location|city) ?",
["I don't have a physical location, as I'm a chatbot. But I'm here to help you with whatever you need!", ]
],
[
r"(.*) (weather|temperature) (in|at) (.*)?",
["You can check the weather at %4 by visiting a weather website or app. Is there anything else I can help you with?", "I'm not sure about the weather at %4, but you can check online to find out. What else can I assist you with today?"]
],
[
r"what (is|are) your (hobbies|interests) ?",
["As a chatbot, I don't have hobbies or interests like humans do. However, I'm always interested in helping you with whatever you need. What can I assist you with today?", ]
],
[
r"(.*) (thanks|thank you) (.*)",
["You're welcome! Is there anything else I can help you with?", "No problem, happy to help. What else can I assist you with today?"]
],
[
r"(.*) (bye|goodbye) (.*)",
["Goodbye! It was nice chatting with you. If you have any more questions or need assistance in the future, don't hesitate to come back and chat with me again.", "Take care, and have a great day!"]
],
[
r"(.*)",
["I'm sorry, I don't quite understand. Can you please rephrase your question or request?", "I'm not sure what you're asking. Can you please clarify or provide more information?"]
]
]
def ada():
print("Hi, I'm Ada. How can I assist you today? \nPlease type lowercase English language to start a conversation. Type 'bye' to end the conversation.")
chat = Chat(QA, reflections)
if __name__ == "__main__":
ada()
chat.converse()
Output
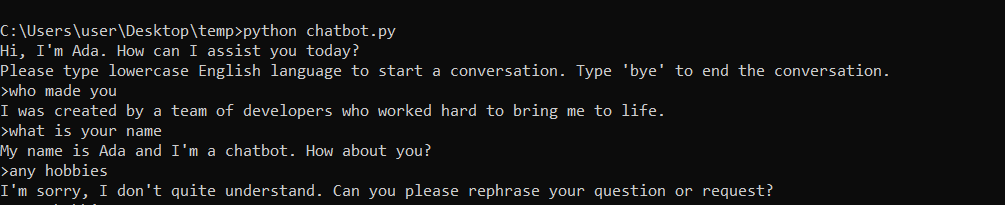
In the above chatbot program, we use the Python NLTK
library to engage in conversation with the user. When you run the program, the chatbot greets you and invites you to start chatting in lowercase English language.
Once you type in a message, the program searches for a matching question from a predefined list and returns an answer.
The chatbot is capable of handling different types of questions such as those related to weather or directions, thanks to the conditional statements in the code.
When you are ready to end the conversation, simply type “quit” and the chatbot will say goodbye.
To ensure that the program only runs when executed directly as a script, we have used the if name == “main“: block. Overall, for a beginner to learn pythons this project provides an opportunity of how NLTK can be used to create a simple chatbot that can respond to user input in a conversational manner.