In this project, you will learn to build a simple calculator in Python. You will use python functions
, while
loop and if-elif
python statement to build a calculator project.
This calculator that you will build is capable of performing basic arithmetic operations such as addition, subtraction, multiplication, and division as shown below.
from prettytable import PrettyTable
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
return x / y
while True:
print('Select operation.')
print('1. Add')
print('2. Subtract')
print('3. Multiply')
print('4. Divide')
print('5. Exit')
choice = input('Enter choice(1/2/3/4/5): ')
if choice == '5':
print('Exiting calculator')
break
num1 = float(input('Enter first number: '))
num2 = float(input('Enter second number: '))
table = PrettyTable(['Operation', 'Result'])
if choice == '1':
result = add(num1, num2)
table.add_row(['Add', result])
print(table)
elif choice == '2':
result = subtract(num1, num2)
table.add_row(['Subtract', result])
print(table)
elif choice == '3':
result = multiply(num1, num2)
table.add_row(['Multiply', result])
print(table)
elif choice == '4':
if num2 == 0:
print('Error: Cannot divide by zero')
else:
result = divide(num1, num2)
table.add_row(['Divide', result])
print(table)
else:
print('Invalid input')
The output of a calculator project
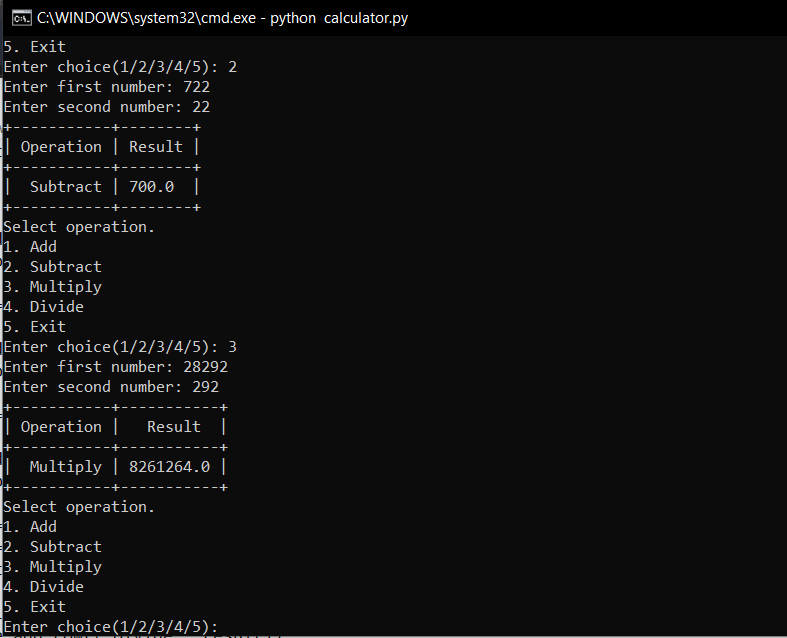