In this article you will learn to create a password manager python project.
A password manager is a useful application that can be built using Python. In this project, you will build a password manager that allows users to securely store and manage their passwords of different websites.
import secrets
import hashlib
import hmac
import sqlite3
from prettytable import PrettyTable
# Connect to the database
conn = sqlite3.connect('passwords.db')
# Create a table to store the passwords
conn.execute('''CREATE TABLE IF NOT EXISTS passwords
(website TEXT NOT NULL, username TEXT NOT NULL, password BLOB NOT NULL);''')
conn.commit()
# Function to encrypt a password
def encrypt_password(password):
salt = secrets.token_bytes(16)
key = hashlib.pbkdf2_hmac('sha256', password.encode('utf-8'), salt, 100000)
return salt + key
# Function to verify a password
def verify_password(password, hashed_password):
salt = hashed_password[:16]
key = hashed_password[16:]
new_key = hashlib.pbkdf2_hmac('sha256', password.encode('utf-8'), salt, 100000)
return hmac.compare_digest(key, new_key)
# Function to add a new password to the database
def add_password():
website = input('Enter website name: ')
username = input('Enter username: ')
password = input('Enter password: ')
hashed_password = encrypt_password(password)
conn.execute("INSERT INTO passwords (website, username, password) VALUES (?, ?, ?)", (website, username, hashed_password))
conn.commit()
print('Password added successfully.')
# Function to retrieve a password from the database
def get_password():
website = input('Enter website name: ')
password = input('Enter password: ')
cursor = conn.execute("SELECT username, password FROM passwords WHERE website=?", (website,))
row = cursor.fetchone()
if row:
username = row[0]
hashed_password = row[1]
if verify_password(password, hashed_password):
print(f'Website: {website}')
print(f'Username: {username}')
print(f'Password: {password}')
else:
print('Invalid password.')
else:
print('Website not found.')
# Function to list all passwords in the database
def list_passwords():
cursor = conn.execute("SELECT website, username, password FROM passwords")
rows = cursor.fetchall()
if len(rows) > 0:
table = PrettyTable(['Website', 'Username', 'Password'])
for row in rows:
website = row[0]
username = row[1]
password = row[2]
table.add_row([website, username, password])
print(table)
else:
print('No passwords found.')
# Main menu loop
while True:
print('1. Add Password')
print('2. Retrieve Password')
print('3. List Passwords')
print('4. Exit')
choice = input('Enter choice: ')
if choice == '1':
add_password()
elif choice == '2':
get_password()
elif choice == '3':
list_passwords()
elif choice == '4':
break
else:
print('Invalid choice. Try again.')
Output while running above program is shown below.
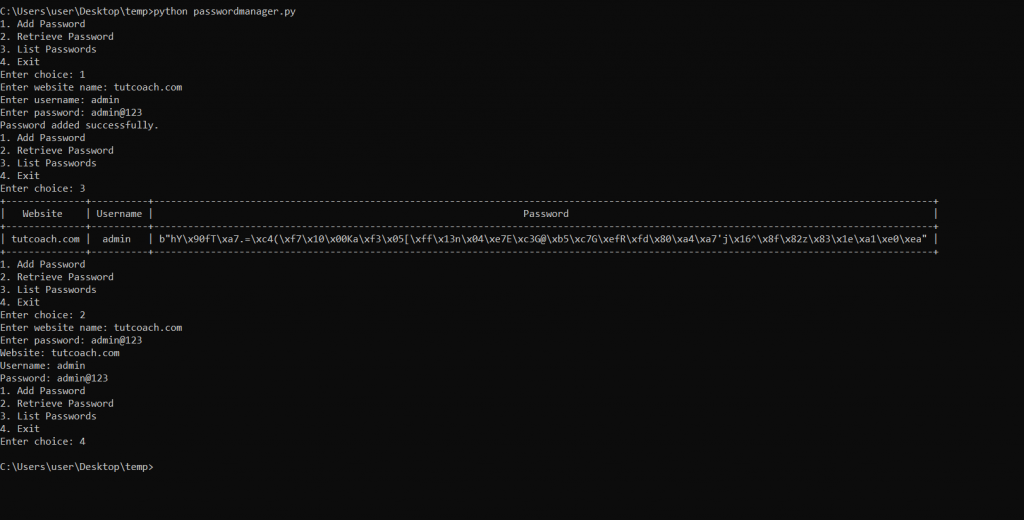
The above password manager project uses the following packages: secrets, hashlib, hmac, and sqlite3
.
The secrets package used here is used to generate a random salt that we use to hash the user’s password using the hashlib.pbkdf2_hmac
function. We also use the hmac
package to compare the hashed password with the user’s input.
We have also used the prettytable
information in tabular format for our users.
To store the passwords securely, we use an SQLite database to encrypt and decrypt the passwords. We create a table called passwords
that has three columns: website, username, and password.
We use the sqlite3 package to create and connect to the database and execute SQL queries.
The program allows the user to add a new password
, list all passwords, and retrieve a password
by website name. We use the input function to get the user’s input and the print function to display messages to the user. We also use the format function to format the output of the list_passwords function in a tabular format.
Overall, the program provides a secure and convenient way to manage passwords for various application.