In this article you will learn to create a hangman game in python.
This classic word-guessing game is a fun and challenging way to test your vocabulary skills. The objective is simple: you have to guess the hidden word by suggesting letters within a limited number of attempts. Each incorrect guess brings you closer to the “hanging” of a stick figure, and if you make too many mistakes, the game is over! But don’t worry, with each correct guess, more of the word is revealed, giving you clues to solve the puzzle. In this Python implementation of Hangman, we’ll generate a random word from a predefined list, and you’ll have a maximum of 6 incorrect guesses before the game ends. Your task is to input single letters as guesses, and the program will let you know if your guess is correct or not. Let’s dive in and see if you can save the stick figure from being “hanged” by guessing the hidden word before running out of attempts!
To start, create a list of words that the game can select from. You can use a text file that contains a list of words or define the list of words in your code. Next, generate a random word from the list of words using the random
module.
Then, create a loop that runs until the player has used up all their attempts or has guessed the word correctly. Inside the loop, ask the player to guess a letter and check if the letter is in the word. If the letter is in the word, update a list that shows the letters that the player has correctly guessed. If the letter is not in the word, decrease the number of attempts remaining.
import random
words = ['apple', 'java']
def get_word():
random_word = random.choice(words)
random_word = list(random_word.upper())
return random_word
def draw_hangman(tries):
hangmanpics = ['''
+---+
| |
|
|
|
|
=========''', '''
+---+
| |
O |
|
|
|
=========''', '''
+---+
| |
O |
| |
|
|
=========''', '''
+---+
| |
O |
/| |
|
|
=========''', '''
+---+
| |
O |
/|\ |
|
|
=========''', '''
+---+
| |
O |
/|\ |
/ |
|
=========''', '''
+---+
| |
O |
/|\ |
/ \ |
|
=========''']
print(hangmanpics[tries])
game_word = get_word()
guessed_word = ['_'] * len(game_word)
tries = 0
# Loop for menu
while True:
# Print menu
print("Welcome to Python Hangman Game!")
print("1. Start a new game")
print("2. Exit game")
# Get user input for menu choice
choice = input("Enter your choice: ")
# Check if choice is valid
if choice not in ['1', '2']:
print("Invalid choice. Please try again.")
continue
# Exit game
if choice == '2':
print("Goodbye!")
break
# Start new game
game_word = get_word()
guessed_word = ['_'] * len(game_word)
tries = 0
# Print initial game state
print("=" * 100)
draw_hangman(tries)
print("Guess this word: ", " ".join(guessed_word))
# Play game
while True:
# Ask for user input
guess = input("Guess a letter: ").upper()
if len(guess) != 1 or not guess.isalpha():
print("Invalid input. Please enter a single letter.")
continue
if guess not in game_word:
print("Incorrect guess.")
tries += 1
draw_hangman(tries)
if tries > 6:
print("You lost the game. The word was:", "".join(game_word))
break
elif guess in game_word:
if guess in guessed_word:
print("You have already guessed this letter. Try another.")
continue
else:
print("Good job!")
for index, letter in enumerate(game_word):
if letter == guess:
guessed_word[index] = letter
print("Word: ", " ".join(guessed_word))
if guessed_word == game_word:
print("Congratulations, you won!")
break
The Demo of the running project is shown below.
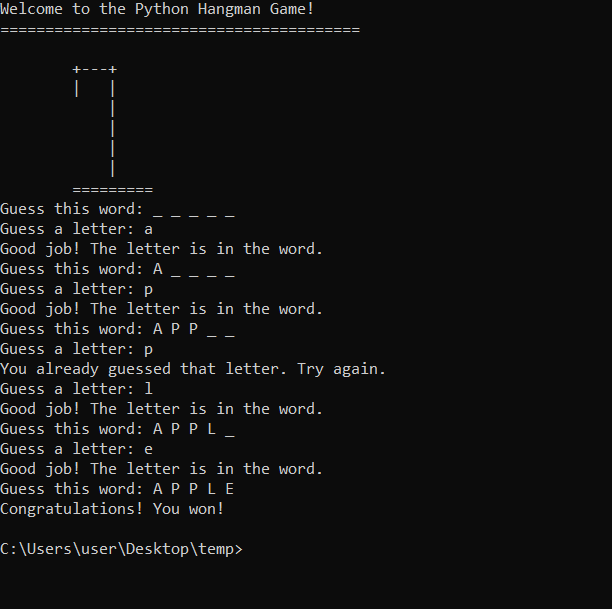
To complete this project First, we need to import a module called “random“. This module will help us choose a random word from a list of words that we will provide. We can add as many words as we want to this list, but for now, we only have two words: “apple” and “java”.
Next, we define two functions: “get_word()” and “draw_hangman(tries)”. The “get_word()” function will choose a random word from our list and convert it to uppercase letters. It will then return this word as a list of letters. The “draw_hangman(tries)” function will help us draw the hangman on the screen based on the number of tries we have.
After we define our functions, we create some variables to keep track of our game progress. We set the game_word
variable to the result of calling the get_word()
function. We set the guessed_word
variable to a list of underscores, one for each letter in the game_word
. We also set the tries
variable to 0 to keep track of how many tries we’ve had so far.
Next, we print some information about the game, including the hangman in its initial state. Then we print the current progress of the game using the guessed_word
variable.
Now, we start a “while” loop that will continue running until the game is won or lost. In each iteration of the loop, we ask the user to guess a letter. If the user’s input is not a single letter or is not a letter at all, we tell them it’s an invalid character and ask them to try again. If the user’s guess is not in the “game_word”, we tell them it’s an incorrect guess, and we increase the “tries” variable. Then we call the “draw_hangman(tries)” function to draw the next part of the hangman based on the number of tries.
If the user’s guess is in the “game_word”, we check if it’s already been guessed before. If it has, we tell the user to try another letter. If it hasn’t been guessed before, we congratulate the user and update the “guessed_word” variable to reflect the new guess. We also print the current progress of the game using the “guessed_word” variable.
Finally, if the “guessed_word” variable is the same as the “game_word” variable, we tell the user that they won the game and break out of the “while” loop. If the “tries” variable is greater than 6, we tell the user that they lost the game and break out of the “while” loop.
So that’s it! We can now play Hangman and have fun.