In this article you will learn to create a Currency Converter python project. A currency converter is a useful project for beginners to learn Python.
In this currency converter project, we use the exchange rate API to convert currency from one currency to another. The project uses the requests module to make a GET request to the API URL and obtain the JSON response.
The program then extracts the rates dictionary from the JSON response, prompting the user to enter the currency codes for the currencies they want to convert and the amount to convert.
The program uses these values and the rates dictionary to compute the converted amount and display the result to the user. The program has a menu option to list all the available currency rates for the USD base currency. This project involves building a currency converter that converts one currency to another based on the current exchange rates.
import requests
url = 'https://api.exchangerate-api.com/v4/latest/USD'
response = requests.get(url)
data = response.json()
rates = data['rates']
def convert_currency():
currency1 = input('Enter currency to convert from: ')
currency2 = input('Enter currency to convert to: ')
amount = float(input('Enter amount to convert: '))
result = amount * (rates[currency2] / rates[currency1])
print(f'{amount} {currency1} is equal to {result} {currency2}')
def list_rates():
for currency, rate in rates.items():
print(f'{currency}: {rate}')
while True:
print("Select an option:")
print("1. Convert currency")
print("2. List all exchange rates")
print("3. Quit")
choice = input()
if choice == "1":
convert_currency()
elif choice == "2":
list_rates()
elif choice == "3":
break
else:
print("Invalid choice")
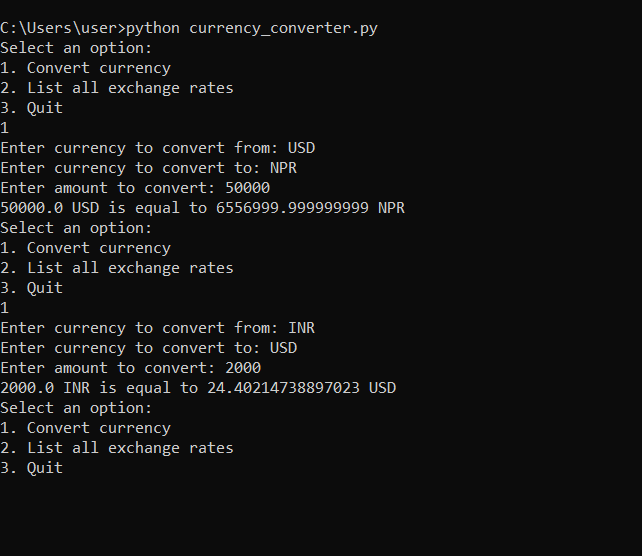