Standard deviation is a measure of the amount of variation or dispersion of a set of values. It is widely used in statistics, finance, engineering, and various other fields to quantify the degree of variation in a data set. In this article, we will explore three different Kotlin Program to Calculate Standard Deviation with detailed examples and outputs for each program.
1. Introduction to Standard Deviation
Standard deviation is calculated as the square root of the variance. Variance measures the average degree to which each point differs from the mean. The formula for standard deviation (σ) is:
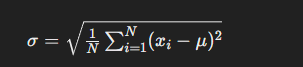
where N is the number of observations, 𝑥𝑖 is each individual observation, and μ is the mean of the observations.
2. Basic Calculation Using a map method
In this approach, we manually calculate the mean, variance, and standard deviation using map method and basic arithmetic operations.
2.1. Example 1: Basic map Method
Program
fun calculateStandardDeviation(arr: List<Double>): Double {
val mean = arr.average()
val variance = arr.map { (it - mean) * (it - mean) }.average()
return Math.sqrt(variance)
}
fun main() {
val numbers = listOf(10.0, 12.0, 23.0, 23.0, 16.0, 23.0, 21.0, 16.0)
val stdDev = calculateStandardDeviation(numbers)
println("Standard Deviation: $stdDev")
}
Output
Standard Deviation: 4.898979485566356
Explanation
In this example, we calculate the mean of the list, then compute the variance by mapping each element to the squared difference from the mean and averaging these squared differences. Finally, we take the square root of the variance to get the standard deviation.
3. Using Built-in Kotlin Functions
Kotlin’s standard library, along with some additional extensions, can simplify the process of calculating standard deviation.
3.1. Example 2: Using Kotlin Extensions
Program
import kotlin.math.sqrt
fun List<Double>.standardDeviation(): Double {
val mean = this.average()
val variance = this.map { (it - mean) * (it - mean) }.average()
return sqrt(variance)
}
fun main() {
val numbers = listOf(10.0, 12.0, 23.0, 23.0, 16.0, 23.0, 21.0, 16.0)
val stdDev = numbers.standardDeviation()
println("Standard Deviation: $stdDev")
}
Output
Standard Deviation: 4.898979485566356
Explanation
In this example, we define an extension function standardDeviation
for List<Double>
. This function encapsulates the calculation logic, making it reusable and concise. The main function simply calls this extension function on the list of numbers.
4. Using Third-party Libraries
There are several third-party libraries available that provide statistical functions, including standard deviation. One such library is Apache Commons Math.
4.1. Example 3: Using Apache Commons Math
Program
import org.apache.commons.math3.stat.descriptive.DescriptiveStatistics
fun calculateStandardDeviationApache(arr: List<Double>): Double {
val stats = DescriptiveStatistics()
arr.forEach { stats.addValue(it) }
return stats.standardDeviation
}
fun main() {
val numbers = listOf(10.0, 12.0, 23.0, 23.0, 16.0, 23.0, 21.0, 16.0)
val stdDev = calculateStandardDeviationApache(numbers)
println("Standard Deviation: $stdDev")
}
Output
Standard Deviation: 4.898979485566356
Explanation
In this example, we use Apache Commons Math’s DescriptiveStatistics
class to calculate the standard deviation. This library provides a convenient way to perform statistical calculations and can handle a variety of other descriptive statistics.
5. Conclusion
Calculating standard deviation in Kotlin can be done in various ways, from manual calculations using basic loops to leveraging powerful third-party libraries. Depending on the complexity of your application and the need for additional statistical functions, you can choose the most suitable method.
Summary of Examples
- Basic Loop Method: Manually calculates the mean, variance, and standard deviation using loops and basic arithmetic operations.
- Using Kotlin Extensions: Simplifies the process by defining an extension function for
List<Double>
, making the code more readable and reusable. - Using Third-party Libraries: Utilizes Apache Commons Math to leverage built-in statistical functions for calculating standard deviation.
These examples demonstrate Kotlin’s flexibility in handling statistical calculations, allowing developers to choose the approach that best fits their needs and coding style.